-
Notifications
You must be signed in to change notification settings - Fork 0
4. JS
Deepak Ramalingam edited this page Feb 18, 2023
·
7 revisions
We will use JS to add code to our pages. Specifically, we will add movie reviews to our database and we will query them from our database. We will also retrieve input from HTML elements.
Good resource to learn JS: https://www.w3schools.com/js/
We will call JavaScript functions from index.html
and rate-movie.html
<!DOCTYPE html>
<html>
<head>
<!-- Title of Webpage (appears in tab name) -->
<title>Spicy Tomatoes</title>
<!-- Link CSS Stylesheet -->
<link rel="stylesheet" href="index.css">
<!-- Fix Scaling of Website on Mobile Devices -->
<meta id="Viewport" name="viewport" content="initial-scale=1, maximum-scale=1, minimum-scale=1, user-scalable=no">
</head>
<body>
<!-- Navbar -->
<div class="navbarContainer">
<img width="50px" height="50px" src="https://d35lsekltq0ycz.cloudfront.net/web-arcade/tomato-transparent.png"/>
<h1>Spicy Tomatoes</h1>
</div>
<!-- Write Review Button -->
<a href="rate-movie.html" class="linkBtn">Review Movie</a>
<!-- List of Ratings for Movies -->
<div class="reviewList" id="reviewList"></div>
<!-- Link JavaScript Code -->
<script type="module" src="index.js"></script>
</body>
</html>
We will implement these functions in index.js
import { getDocsFromCollection } from "./dao.js";
// get list container for reviews
const reviewList = document?.getElementById("reviewList");
/**
* Generate the HTML string for a movie review
* @param {string} movieName
* @param {string} reviewText
* @returns renderable HTML string for a movie review list item
*/
function generateReviewHTML(movieName, reviewText) {
return (`
<div class="reviewContainer">
<p class="movieName">${movieName || ""}</p>
<p class="reviewText">${reviewText || ""}</p>
</div class="reviewContainer">
`);
}
/**
* Fetch and render reviews from database
*/
function fetchReviews() {
if (reviewList) {
// clear content of review list
reviewList.innerHTML = "";
getDocsFromCollection("reviews")
.then((reviews) => {
reviews.forEach((review) => {
const movieName = review?.data()?.movieName;
const reviewText = review?.data()?.reviewText;
reviewList.innerHTML += generateReviewHTML(movieName, reviewText);
});
})
.catch((err) => {
console.error("Unable to fetch reviews from database", err);
alert("Error fetching reviews from database");
});
}
}
// when window loads, attempt to update review list
window.addEventListener("load", fetchReviews, false);
<!DOCTYPE html>
<html>
<head>
<!-- Title of Webpage (appears in tab name) -->
<title>Spicy Tomatoes</title>
<!-- Link CSS Stylesheet -->
<link rel="stylesheet" href="index.css">
<!-- Fix Scaling of Website on Mobile Devices -->
<meta id="Viewport" name="viewport" content="initial-scale=1, maximum-scale=1, minimum-scale=1, user-scalable=no">
</head>
<body>
<!-- Navbar -->
<div class="navbarContainer">
<img width="50px" height="50px" src="https://d35lsekltq0ycz.cloudfront.net/web-arcade/tomato-transparent.png"/>
<h1>Spicy Tomatoes</h1>
</div>
<!-- Form for Typing and Submitting Review -->
<div class="reviewFormContainer">
<input placeholder="movie name" type="text" id="movieNameInput"/>
<input placeholder="review" type="text" id="reviewInput"/>
<button id="submitReviewBtn">Submit</button>
</div>
<!-- Continue to Ratings Page Button -->
<a href="index.html" class="linkBtn">Back</a>
<!-- Link JavaScript Code -->
<script type="module" src="rate-movie.js"></script>
</body>
</html>
We will implement these functions in rate-movie.js
import { addDocToCollection } from "./dao.js";
// get submit review button
const submitReviewBtn = document?.getElementById("submitReviewBtn");
// get movie name input field
const movieNameInput = document?.getElementById("movieNameInput");
// get review input field
const reviewInput = document?.getElementById("reviewInput");
/**
* Add review to database
*/
function submitReview() {
if (movieNameInput && reviewInput) {
const movieName = movieNameInput?.value;
const reviewText = reviewInput?.value;
if (movieName && reviewText) {
addDocToCollection({ movieName, reviewText }, "reviews")
.then(() => {
window.location.href = 'index.html';
}).catch((err) => {
console.error("Unable to create new review in database", err);
alert("Error creating review in database");
});
} else {
alert("Please fill all input fields.");
}
}
}
// add on click listener to submit review button
if (submitReviewBtn) {
submitReviewBtn.onclick = function() {
submitReview();
};
}
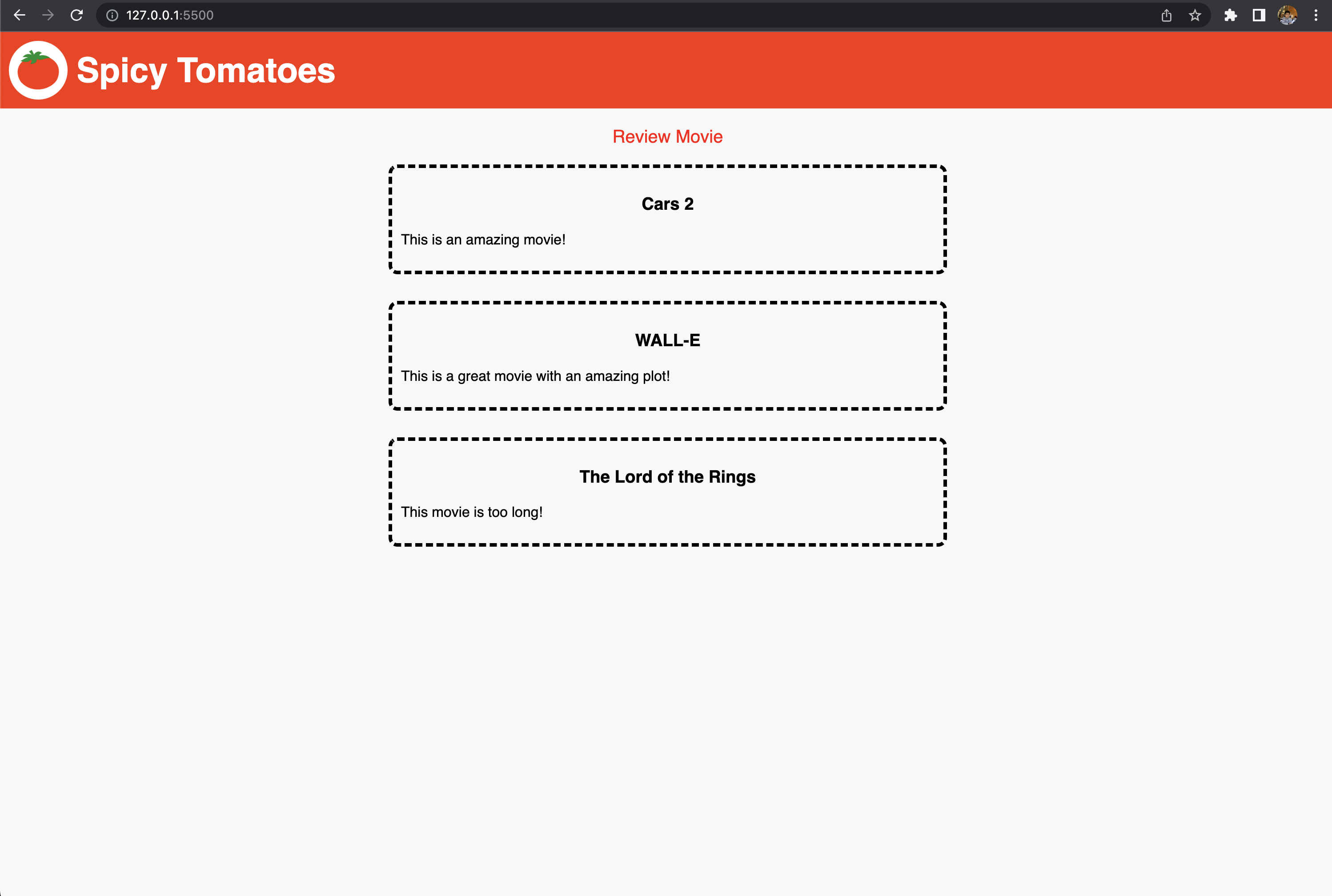
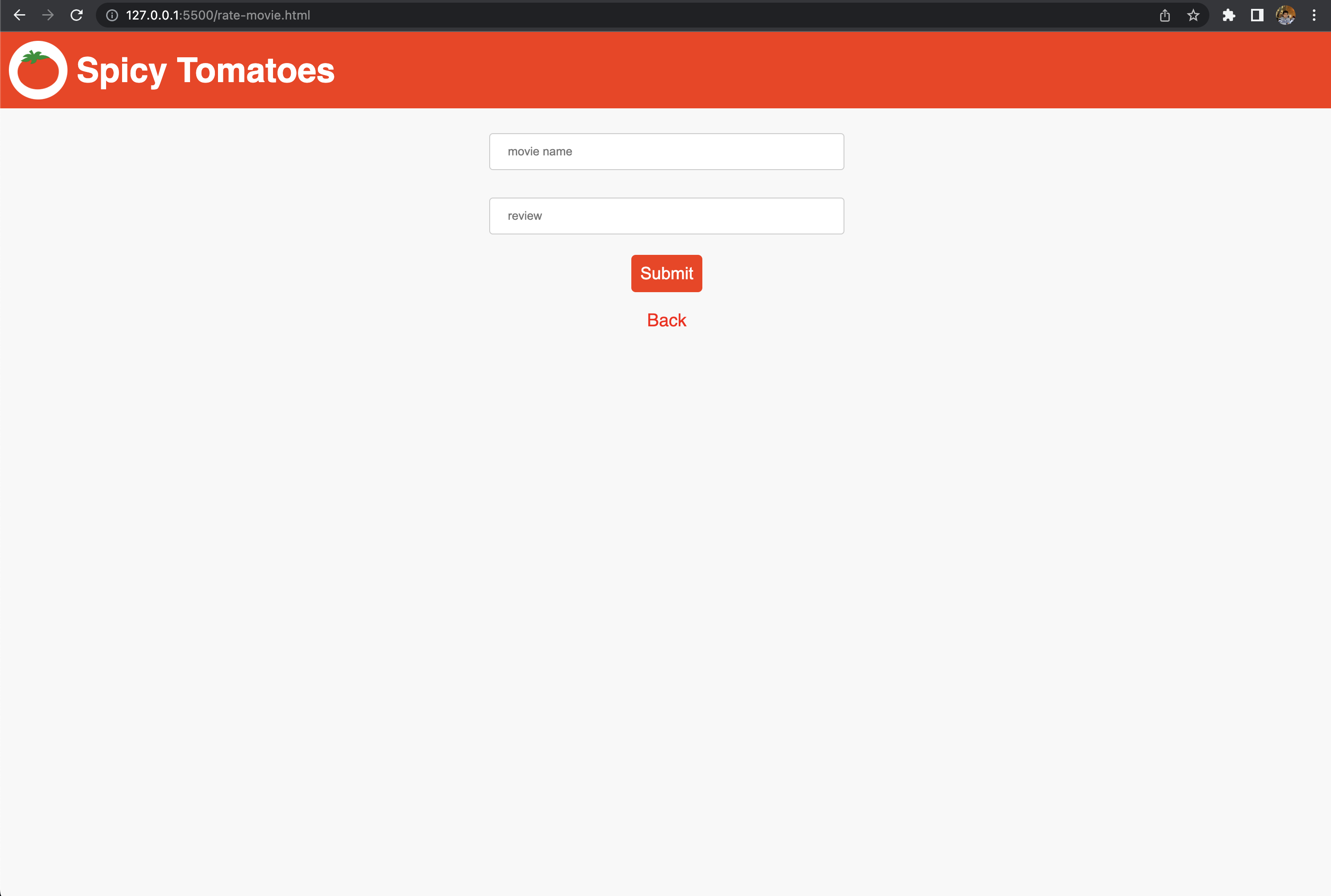